Ruby on Rails & Clean Code
My latest programming adventures have been reading “Clean Code” by Robert C. Martin and working on a web app using Ruby on Rails.
Clean Code
I’ve always had the problem that I knew my code looked bad but wasn’t quite sure how to improve it beyond some basic formatting and renaming.
So I got “Clean Code” to guide me, as I found it very highly reviewed online for my exact problem.
Now I’ve only read so far page 116 out of 409 readable pages but here are my thoughts on the book.
Thoughts:
Clean code does a fantastic job of giving you plenty of examples of good and bad code and how to improve the look of your code. Covering formatting, naming, comments, functions and more.
There is plenty in the book that is going over my head as a novice coder but that just means it is opening up my mind to more topics. It is a very enjoyable and well written book also which was a surprise.
Recommendation:
I think if you are interested in improving your code “smell” it is worth buying or finding a free copy somewhere.
Ruby on Rails - Web App
I’m going to give a brief rundown of the project and then some starter solutions to issues that you might bump into like I did.
My Ruby on Rails college project was to make a single page website where a user signup/in and could add candidates and then add skills to those candidates. So they had a one to many relationship. To me it would make more sense to me to have a many to many relationship with an entity in between, but I don’t write the questions.
This the basic sketch I made to start off my project. I find even a basic sketch a big help to start off in the right direction. The idea is three tabs which can cover all the required functions.
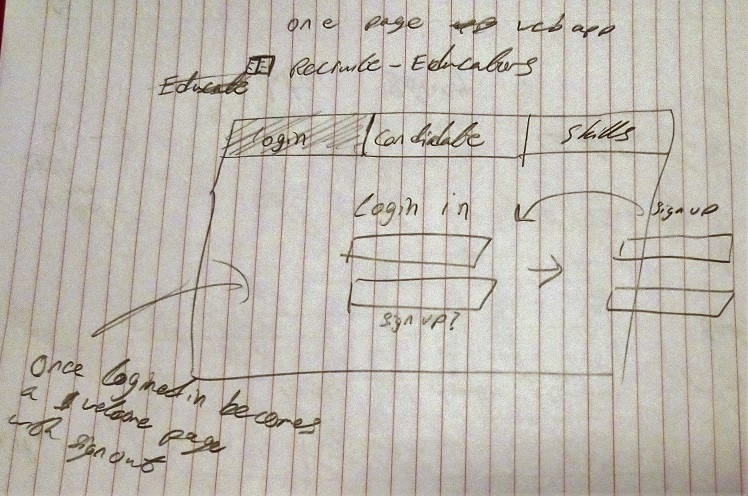
This is the completed page for the site.
I used bootstrap to make a Nav tab so you can easily switch between the views.
In each tab a render is called from a partial to fill these tabs as shown in the code below.
index.html.erb
<div class="tab-content" id = "maintable" >
<div class="tab-pane active text-center" id="login" role="tabpanel" aria-labelledby="login-tab">
<%= render "loginTab" %>
</div>
<div class="tab-pane" id="candidates" role="tabpanel" aria-labelledby="candidates-tab">
<div id = "rencandidates">
<%= render "candidateTab" %>
</div>
</div>
<div class="tab-pane" id="skillstab1" role="tabpanel" aria-labelledby="skills-tab">
<div id = "renskills">
<%= render "skillsTab" %>
</div>
</div>
</div>
Below is a view of the modals I created to allow the user to create new/edit/show objects. This again is using more bootstrap this time their modals.
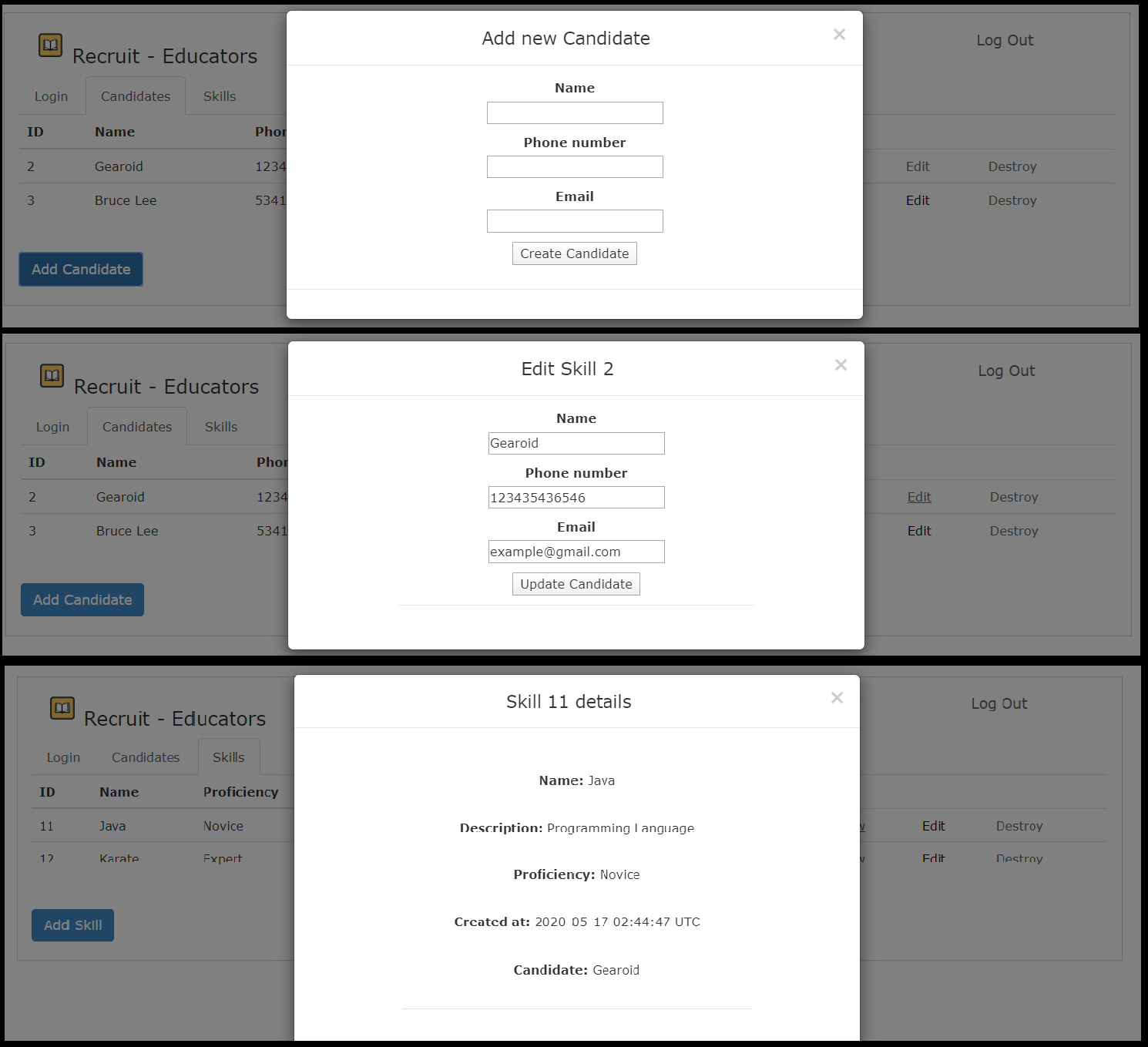
Anything with <%# %> in html.erb files are comments
Below is the code that produces a modal when the “Add Candidate” button is pressed. Within it calls a render for a fresh candidate’s form details to be placed inside the modal.
_candidateTab.html.erb
<div id="myModal" class="modal fade" role="dialog">
<div class="modal-dialog">
<div class="modal-content text-center">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal">×</button>
<h4 class="modal-title">Add new Candidate</h4>
</div>
<%# Errors get inserted using javascript %>
<div id = "candidateerrors"></div>
<div class="modal-body div-centered">
<%= render 'candidates/form', candidate: Candidate.new %>
</div>
<div class="modal-footer">
</div>
</div>
</div>
</div>
Project Issues
1. Keeping the same tab open upon page refresh
For this issue I found some helpful javascript on stack overflow. This code puts the tab id into the url and reads it from there the tab can be kept track.
home.js
$(document).ready(function() {
if (location.hash) {
$("a[href='" + location.hash + "']").tab("show");
}
$(document.body).on("click", "a[data-toggle='tab']", function(event) {
location.hash = this.getAttribute("href");
});
});
$(window).on("popstate", function() {
var anchor = location.hash || $("a[data-toggle='tab']").first().attr("href");
$("a[href='" + anchor + "']").tab("show");
});
2. Modal fade not disappearing
The solution I found was making a coffeescript call that simply removed the modal backdrop. I also ended up having to refresh the page as my skills weren’t getting the updated candidates to pick from (not Ideal).
There is also an error check, that inserts a render of a shared error message.
create.js.coffee
<% if @candidate.errors.any? %>
$("#candidateerrors").html("<%= escape_javascript(render "home/error_message", object: @candidate) %>");
<% else %>
$ location.reload();
$(".modal-backdrop").remove();
/* Renders the candidate tab in a div */
$("#rencandidates").html("<%= escape_javascript(render "home/candidateTab") %>");
<% end %>
Below is the controller method I used to call create.js.coffee just to give you a bit more of the picture.
candidates_controller.rb
def create
@candidate = Candidate.new(candidate_params)
respond_to do |format|
if @candidate.save
format.html { redirect_to root_url }
format.js
format.json { render :show, status: :created, location: @candidate }
else
format.js { render layout: false, content_type: 'text/javascript' }
format.html
end
end
end
Drop me a message on Twitter if you want some help doing something similar.
Have a nice day good luck, happy coding!